"|  | \"When you want to feel like a rebel, but your bike is still in the shop. 🏍️😂\" |\n",
"| 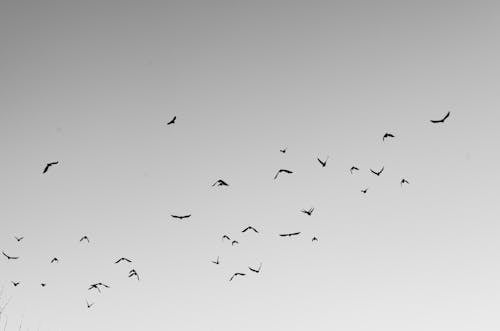 | \"When the squad is finally ready to leave the party but you can't find your keys. 🕊️🤣\" |\n",
"| 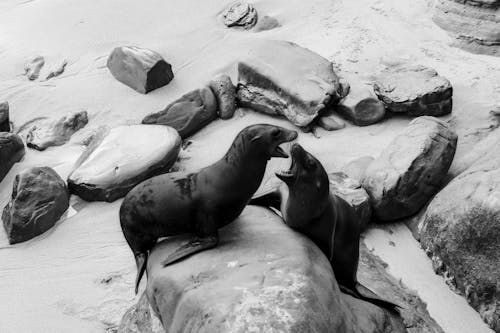 | \"When you’re trying to chill at the beach, but your buddy won’t stop splashing you. 🦭💦\" |\n",
"```\n",
"\n",
"Feel free to use or modify the layout and the tweets as you see fit!"
],
"text/plain": [
"<IPython.core.display.Markdown object>"
]
},
"metadata": {},
"output_type": "display_data"
}
],
"source": [
"source": [
"# A small exercise to feed the llm with image alt text and return a funny tweet.\n",
"\n",
"# Step 1: Create your prompts\n",
"# Step 1: Create your prompts\n",
"import json\n",
"system_prompt = \"You are a meme lord. You like tweeting funny and hilarious comments on images. To understand the image you would be given alt text on the image.\"\n",
" self.image_urls = [img['src'] for img in image_tags if img.get('src')]\n",
" self.image_alt = [img['alt'] if img.get('alt') else \"\" for img in image_tags]\n",
"\n",
" # Restricting to 3 images only.\n",
" if self.image_urls:\n",
" self.images = {self.image_urls[i]:self.image_alt[i] for i in range(4)}\n",
" else:\n",
" self.images = {}\n",
" \n",
" \n",
"system_prompt = \"something here\"\n",
"user_prompt = \"\"\"\n",
" Lots of text\n",
" Can be pasted here\n",
"\"\"\"\n",
"\n",
"\n",
"# Step 2: Make the messages list\n",
"def user_prompt_for(website):\n",
" user_prompt = f\"Following are images with their alt-text:\"\n",
" user_prompt += json.dumps(website.images)\n",
" user_prompt += \"\\n Give me a markdown layout with tables for each image where each image is given its own row, with the image itself on the left and funny tweet on the right.\"\n",
" return user_prompt\n",
"\n",
"\n",
"messages = [] # fill this in\n",
"\n",
"\n",
"# Step 3: Call OpenAI\n",
"# Step 2: Make the messages list\n",
"page = website(\"https://www.pexels.com/\")\n",
"user_prompt = user_prompt_for(page)\n",
"messages = [{\"role\":\"system\",\"content\":system_prompt},{\"role\":\"user\", \"content\":user_prompt}] # fill this in\n",